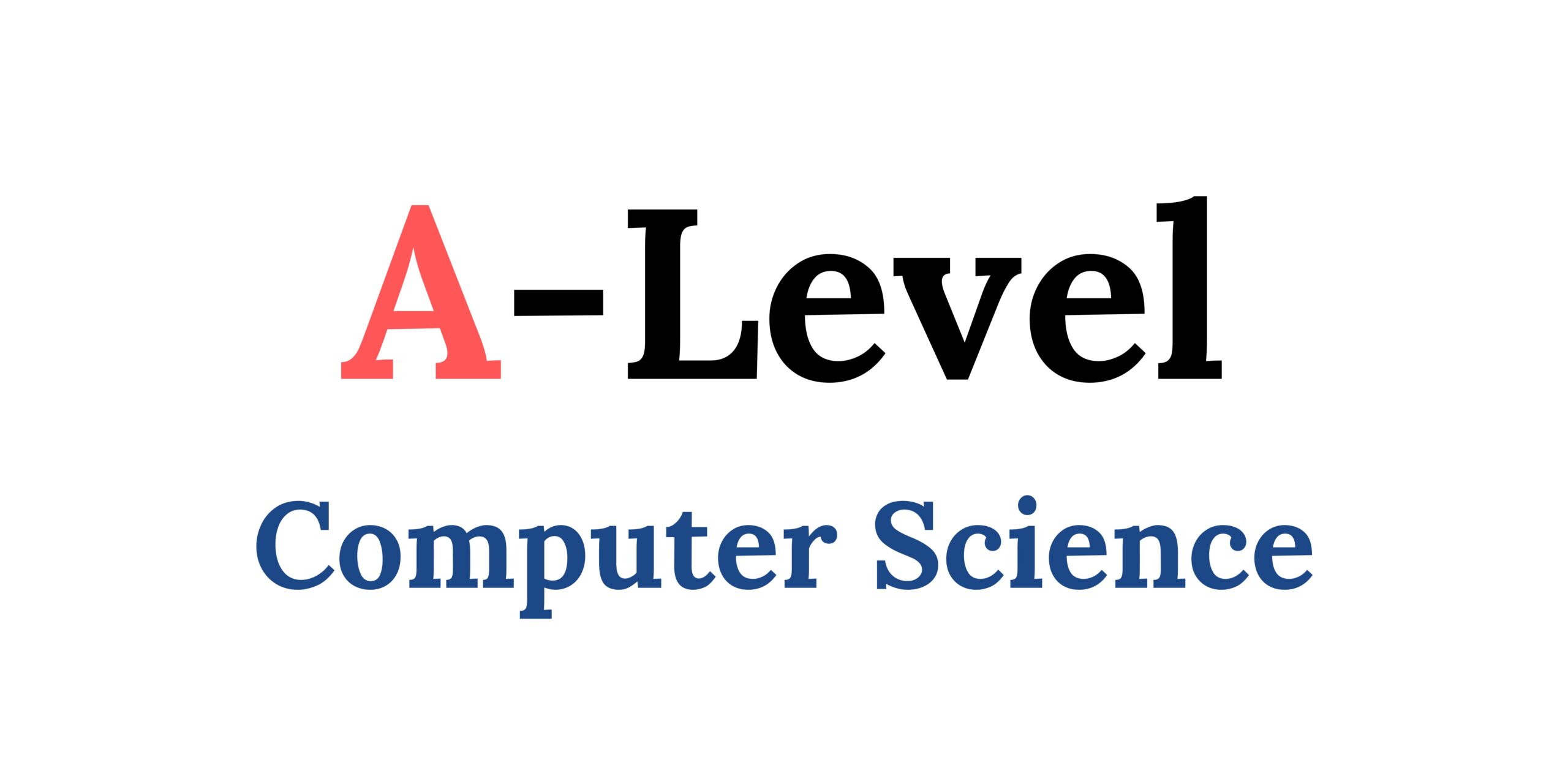
Overview of AQA A-level Computer Science qualifications
Subject content:
Want to learn more about Advanced Level Qualifications (A-Levels) and how they can shape your academic future? Click here to explore: A-Level Information.
1. Fundamentals of programming
Programming
Scope of study | Content |
---|---|
Data types | • Understand the concept of data types. • Identify and use: integer, float, Boolean, character, string, date/time, pointer/reference, records, and arrays. • Define and use custom data types based on built-in types. |
Programming concepts | • Combine statement types: declarations, assignment, iteration, selection, and subroutines. • Use loops (definite, indefinite, nested) with start or end conditions. • Apply nested selection. • Use meaningful identifiers. |
Arithmetic operations in a programming language | Use and understand: addition, subtraction, multiplication, float division, integer division (with remainders), exponentiation, rounding, and truncation. |
Relational operations in a programming language | Understand and use: equal to, not equal to, less than, greater than, less than or equal to, and greater than or equal to. |
Boolean operations in a programming language | Be familiar with and be able to use: NOT, AND, OR, XOR. |
Constants and variables in a programming language | Explain the difference between variables and constants and the benefits of using named constants. |
String-handling operations in a programming language | Understand and use: length, position, substring, concatenation, character-code conversions, and string conversions. |
Random number generation in a programming language | Be familiar with, and be able to use, random number generation. |
Exception handling | Understand the concept and usage of exception handling in a familiar programming language. |
Subroutines (procedures/functions) | Understand subroutines as named blocks of code callable by name. Explain their advantages in programming. |
Parameters of subroutines | Understand the use of parameters to pass data and use subroutines with interfaces. |
Returning a value/values from a subroutine | Use subroutines that return values to the calling routine. |
Local variables in subroutines | Subroutines can declare local variables, which exist only during execution and are accessible within the subroutine. Use them and explain their benefits. |
Global variables in a programming language | Be able to contrast local variables with global variables. |
Role of stack frames in subroutine calls | Understand how stack frames store return addresses, parameters, and local variables during subroutine calls. |
Recursive techniques | Understand recursive techniques, including base and general cases, and their implementation. Solve simple problems using recursion. |
Programming paradigms
Scope of study | Content |
---|---|
Programming paradigms | Understand the characteristics of procedural and object-oriented programming, with experience in both. |
Procedural-oriented programming | • Understand structured program design and construction. • Use hierarchy charts in program design. • Explain the advantages of a structured approach. |
Object-oriented programming | • Understand concepts: class, object, instantiation, encapsulation, inheritance, aggregation, composition, polymorphism, overriding. • Know the benefits of OOP and design principles: – Encapsulate what varies – Favor composition over inheritance – Program to interfaces, not implementation. • Write object-oriented programs and interpret class diagrams. |
2. Fundamentals of data structures
Scope of study | Content |
---|---|
Data structures and abstract data types | • Data structures • Single- and multi-dimensional arrays (or equivalent) • Fields, records and files • Abstract data types/data structures |
Queues | Describe and apply operations to linear, circular, and priority queues: – Add an item – Remove an item – Test for empty or full queue. |
Stacks | Describe and apply stack operations: – Push – Pop – Peek or top – Test for empty or full stack. |
Graphs | • Understand graphs for complex relationships and common uses. Know terms: Graph, weighted graph, vertex/node, edge/arc, undirected, and directed. • Compare adjacency matrices and lists for graph representation. |
Trees | • Understand a tree as a connected, undirected graph with no cycles. • Know that a rooted tree has a designated root, with parent-child relationships, and all nodes are descendants of the root. • Understand a binary tree as a rooted tree where each node has at most two children. • Be familiar with typical uses for rooted trees. |
Hash tables | • Understand hash tables and their uses. • Apply simple hashing algorithms. • Know what collisions are and how rehashing handles them. |
Dictionaries | • Understand the concept of a dictionary and its simple applications, such as information retrieval. • Have experience using a dictionary data structure in programming. |
Vectors | • Understand vector notations: – Example: [2.0, 3.14159, -1.0, 2.718281828] – ℝ⁴, function interpretation (e.g., 0 ↦ 2.0). • Represent vectors with dictionary, list, or 1-D array. • Visualize as arrows. • Know vector addition, scalar multiplication, convex combinations, and dot product applications. |
3. Fundamentals of algorithms
Scope of study | Content |
---|---|
Graph-traversal | Trace breadth-first and depth-first search algorithms and understand their typical applications. |
Tree-traversal | • Trace pre-order, post-order, and in-order tree traversal algorithms. • Understand their uses. |
Reverse Polish | • Convert simple infix expressions to Reverse Polish notation (RPN) and vice versa. • Understand its uses and advantages. |
Searching algorithms | • Linear search • Binary search • Binary tree search |
Sorting algorithms | • Bubble sort • Merge sort |
Optimisation algorithms | • Understand and trace Dijkstra’s algorithm. • Know its applications in shortest path problems. |
4. Theory of computation
Scope of study | Content |
---|---|
Abstraction and automation | • Problem-solving • Following and writing algorithms • Abstraction • Information hiding • Procedural abstraction • Functional abstraction • Data abstraction • Problem abstraction/reduction • Decomposition • Composition • Automation |
Regular languages | • Finite state machines (FSMs) with and without output • Maths for regular expressions • Regular expressions • Regular language |
Context-free languages | • Check language syntax using BNF or syntax diagrams and create simple production rules. • Understand why BNF can represent languages that regular expressions cannot. |
Classification of algorithms | • Comparing algorithms • Maths for understanding Big-0 notation • Order of complexity • Limits of computation • Classification of algorithmic problems • Computable and non-computable problems • Halting problem |
A model of computation | • Understand Turing machines for simple computations, with components: – Finite states, alphabet, infinite tape, and read-write head. – Start and halting states. • Know the equivalence between transition functions and state diagrams. • Be able to represent and trace simple Turing machines. • Understand the importance of Turing machines and the Universal Turing machine in computation. |
5. Fundamentals of data representation
Scope of study | Content |
---|---|
Number systems | • Be familiar with the concept of a natural number and the set ℕ of natural numbers (including zero). • Integer numbers • Rational numbers • Irrational numbers • Real numbers • Ordinal numbers • Counting and measurement |
Number bases | • Understand number bases: decimal (10), binary (2), and hexadecimal (16). • Convert between decimal, binary, and hexadecimal. • Use hexadecimal as a binary shorthand and know why it is used. |
Units of information | • Bits and bytes • Understand binary prefixes (powers of 2): Kibi (Ki) = 2¹⁰, Mebi (Mi) = 2²⁰, Gibi (Gi) = 2³⁰, Tebi (Ti) = 2⁴⁰. • Understand decimal prefixes (powers of 10): Kilo (k) = 10³, Mega (M) = 10⁶, Giga (G) = 10⁹, Tera (T) = 10¹². |
Binary number system | • Unsigned binary • Unsigned binary arithmetic • Signed binary using two’s complement • Numbers with a fractional part • Rounding errors • Absolute and relative errors • Range and precision • Normalisation of floating point form • Underflow and overflow |
Information coding systems | • Character form of a decimal digit • ASCII and Unicode • Error checking and correction |
Representing images, sound and other data | • Bit patterns, images, sound and other data • Analogue and digital • Analogue/digital conversion • Bitmapped graphics • Vector graphics • Vector graphics versus bitmapped graphics • Digital representation of sound • Musical Instrument Digital Interface (MIDI) • Data compression • Encryption |
6. Fundamentals of computer systems
Scope of study | Content |
---|---|
Hardware and software | • Relationship between hardware and software • Classification of software • System software • Role of an operating system (OS) |
Classification of programming languages | • Low-level: Machine code, assembly language. • High-level: Imperative languages. Understand and compare their features, uses, and relationships. |
Types of program translator | • Understand the role of each of the following: assembler, compiler, interpreter. • Distinguish between compilation and interpretation. • Understand the difference between source code and object (executable) code. |
Logic gates | • Construct truth tables for: NOT, AND, OR, XOR, NAND, NOR. • Draw, interpret, and trace logic gate circuits, including half-adders and full-adders. • Complete truth tables for given circuits and write Boolean expressions. • Convert Boolean expressions into equivalent circuits. • Understand and construct a half-adder circuit. • Recognize the edge-triggered D-type flip-flop as a memory unit. |
Boolean algebra | Apply Boolean identities and De Morgan’s laws to simplify Boolean expressions. |
7. Fundamentals of computer organisation and architecture
Scope of study | Content |
---|---|
Internal hardware components of a computer | • Understand basic computer components and their roles: Processor, main memory, address bus, data bus, control bus, I/O controllers. • Know how components communicate via buses (address, data, control). • Explain and compare von Neumann and Harvard architectures and their applications. |
The stored program concept | Be able to describe the stored program concept. |
Structure and role of the processor and its components | • The processor and its components • The Fetch-Execute cycle and the role of registers within it • The processor instruction set • Addressing modes • Machine-code/assembly language operations • Interrupts • Factors affecting processor performance |
External hardware devices | • Input and output devices • Secondary storage devices |
8. Consequences of uses of computing
Individual (moral), social (ethical), legal and cultural issues and opportunities
Understand the impacts of computing on society:
– Transformed communication, monitoring, and data analysis capabilities.
– Responsibility of computer scientists for the ethical design and use of algorithms.
– Software embeds moral and cultural values, influencing global scale outcomes.
– Balancing benefits and risks, including potential harm.
Discuss the challenges of creating legislation in the digital age.
9. Fundamentals of communication and networking
Scope of study | Content |
---|---|
Communication | • Communication methods • Communication basics |
Networking | • Network topology • Types of networking between hosts • Wireless networking |
The Internet | • The Internet and how it works. Structure, role of packet switching and routers, basic packet components, terms (FQDN), “domain name” and “IP address”. • Internet security |
The Transmission Control Protocol/Internet Protocol (TCP/IP) protocol | • TCP/IP • Standard application layer protocols • IP address structure • Subnet masking • IP standards • Public and private IP addresses • Dynamic Host Configuration Protocol (DHCP) • Network Address Translation (NAT) • Port forwarding • Client server model • Thin- versus thick-client computing |
10. Fundamentals of databases
Scope of study | Content |
---|---|
Conceptual data models and entity relationship modelling | • Create a data model based on given requirements for a simple scenario with multiple entities. • Produce entity relationship diagrams (ERDs) and describe entities using the format: Entity1 (Attribute1, Attribute2, …). |
Relational databases | • Explain the concept of a relational database. • Be able to define the terms: attribute, primary key, composite primary key, foreign key. |
Database design and normalisation techniques | • Normalize relations to third normal form (3NF). • Understand that normalization reduces redundancy and ensures data integrity. |
Structured Query Language (SQL) | • Retrieve, update, insert, and delete data from multiple tables using SQL. • Define a database table using SQL. |
Client server databases | Know how concurrent access can be controlled to preserve the integrity of the database. |
11. Big Data
• Big Data refers to data too large for traditional storage systems and is characterized by:
– Volume: Data too large for a single server.
– Velocity: Real-time or near-real-time data streaming.
– Variety: Diverse data types (structured, unstructured, text, multimedia).
• Large data processing requires distributed systems across multiple machines, with functional programming aiding in writing correct and efficient distributed code.
• Graph schema: Represents dataset structure using nodes, edges, and properties.
• Fact-based model: Used for data representation.
12. Fundamentals of functional programming
Scope of study | Content |
---|---|
Functional programming paradigm | • Function type • First-class object • Function application • Partial function application • Composition of functions |
Writing functional programs | • Experience in constructing simple programs in a functional programming language. • Understanding and using higher-order functions. • Experience with functional programming functions like: – map – filter – reduce (or fold) |
Lists in functional programming | A list consists of a head (element) and a tail (list), which can be empty. • Key operations: – Return head/tail, test for empty, return length – Construct, prepend, or append items to a list • Experience writing programs for these operations in a functional or functional-supporting language. |
13. Systematic approach to problem solving
• Analysis: Define the problem, establish system requirements through user interaction, and create a data model. This process may involve prototyping/agile approaches.
• Design: Plan the solution, including data structures, algorithms, modular structure, and user interfaces. Design can be iterative, often using prototyping/agile methods.
• Implementation: Convert models and algorithms into data structures and code. This may be done iteratively, focusing on solving critical issues first.
• Testing: Test the implementation with normal, boundary, and erroneous data, and conduct acceptance testing with the user to ensure the solution meets the specification.
• Evaluation: Assess the computer system based on predefined criteria.
14. Non-exam assessment – the computing practical project
Purpose
The project allows students to develop practical skills by solving a realistic problem or conducting an investigation. It serves as both a learning experience and an assessment, focusing on the ability to create a programmed solution. 42 out of 75 marks are for the technical solution, with the remaining marks for concise supporting documentation.
Types of Problems/Investigations
Students should select a problem of interest within a field they are familiar with. They should ensure that the problem allows them to demonstrate their technical skills. Examples include:
– Simulations (business, scientific, Game of Life)
– Data processing solutions (membership systems)
– Optimization problems (rota creation, route finding)
– Computer games, AI applications
– Control systems (Arduino)
– Dynamic websites, mobile apps
– Data science or machine learning investigations
Problems should be sufficiently different within the same center to avoid overlap. Teachers must confirm this on the Candidate Record Form.
If you need help with Computer Science or any other subject, our tutors are ready to support you on your academic journey. Don’t miss your chance to succeed—take a trial lesson today!
Assessment
Component | Content | Questions | Final score | Weighting of final grade |
---|---|---|---|---|
Paper 1 | This paper assesses a student’s programming skills and theoretical knowledge of Computer Science, covering subject content from sections 1-4 and the skills from section 13. | Students will answer short questions and write, adapt, or extend programs in an Electronic Answer Document provided. Preliminary Material, including a Skeleton Program in various programming languages and test data (where applicable), will be issued for use in the exam. | 100 marks | 40% of A-level |
Paper 2 | This paper tests the student’s ability to answer questions from course content 5-12 above. | Compulsory short-answer and extended-answer questions. | 100 marks | 40% of A-level |
Non-exam assessment | The extra-exam assessment assesses the student’s ability to use the knowledge and skills acquired in the course to solve or investigate a practical problem. Students are expected to follow a systematic approach to problem solving as outlined in section 13 above. | 75 marks | 20% of A-level |
Weighting of assessment objectives for A-level Computer Science
Exams will assess students on the following objectives:
AO1: Knowledge and understanding of computer science principles, including abstraction, logic, algorithms, and data representation.
AO2: Application of knowledge to analyze problems computationally.
AO3: Design, program, and evaluate computer systems, making reasoned judgments and presenting conclusions.
Assessment objectives AOs* | Paper 1 (%) | Paper 2 (%) | NEA (%) | Overall Weighting (%) |
---|---|---|---|---|
AO1 | 8 | 22 | 0 | 30 |
AO2 | 12 | 16 | 2 | 30 |
AO3 | 20 | 2 | 18 | 40 |
Overall weighting of components | 40 | 40 | 20 | 100 |
Assessment weightings
Marks from exam papers will be adjusted to match the component weightings. The final mark is the sum of these scaled marks, and grade boundaries will be determined based on the total scaled mark.
Сomponent | Maximum raw mark | Scaling factor | Maximum scaled mark |
---|---|---|---|
Paper 1 | 100 | ×1.5 | 150 |
Paper 2 | 100 | ×1.5 | 150 |
NEA | 75 | ×1 | 75 |
Total scaled mark: | 375 |